Mastering RStudio: From Sample R Script to Interactive RShiny App
Learning RStudio can be a game-changer for those looking to dive into data analysis and visualization. This powerful tool offers a range of functionalities that can help you transform raw data into meaningful insights. In this post, we'll guide you through the process of mastering RStudio, from writing a sample R script to creating an interactive RShiny app.
Getting Started with RStudio
Before you start, ensure you have R and RStudio installed on your computer. RStudio provides an integrated development environment (IDE) that makes it easier to write, debug, and visualize R code. Begin by familiarizing yourself with the interface, which includes panes for the script editor, console, environment, and plots.
Start by writing a simple R script. Open a new script file and type the following code:
# Sample R Script
data <- mtcars
summary(data)
plot(data$mpg, data$hp)
This script loads the built-in mtcars dataset, provides a summary of the data, and creates a scatter plot of miles per gallon (mpg) against horsepower (hp).
Understanding Data Manipulation
Data manipulation is a key skill in data analysis. R offers several packages like dplyr and tidyr that simplify data manipulation tasks. For example, you can use dplyr to filter, select, and arrange data efficiently. Here's a quick example:
library(dplyr)
filtered_data <- data %>%
filter(mpg > 20) %>%
select(mpg, hp, wt) %>%
arrange(desc(mpg))
This code filters the mtcars dataset for cars with mpg greater than 20, selects relevant columns, and arranges the data in descending order of mpg.
Creating Visualizations
Visualization is a powerful way to communicate data insights. RStudio supports various visualization libraries like ggplot2, which allows you to create complex and aesthetically pleasing charts. Here's an example of a simple bar plot:
library(ggplot2)
ggplot(data, aes(x = factor(cyl), fill = factor(cyl))) +
geom_bar() +
labs(title = "Number of Cars by Cylinder", x = "Cylinders", y = "Count")
This code creates a bar plot showing the number of cars by the number of cylinders. The ggplot2 package offers extensive customization options to enhance your visualizations.
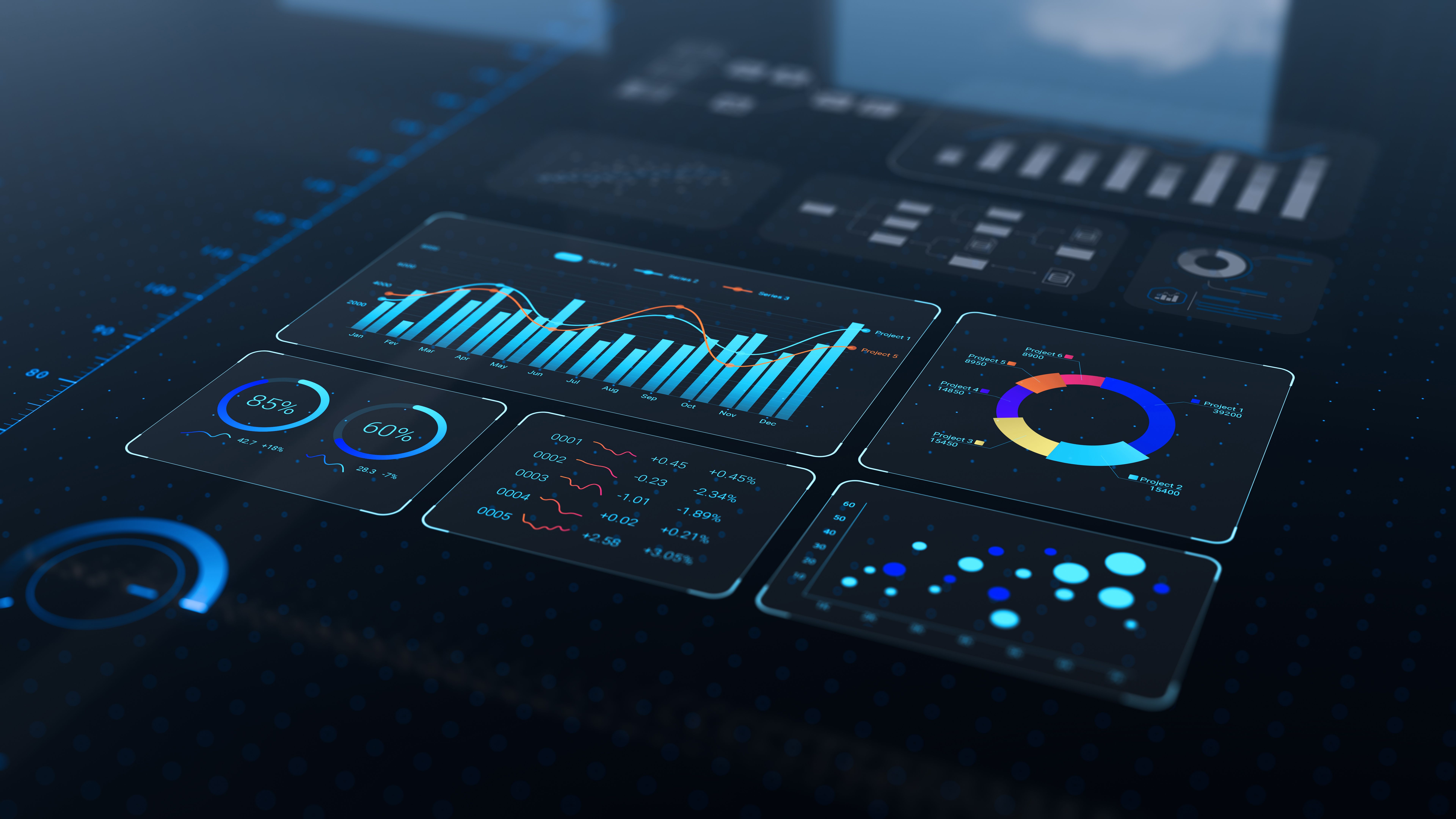
Introduction to RShiny
RShiny is a package that allows you to build interactive web applications using R. These apps can be used to share your data analysis and visualizations with others. To create a basic RShiny app, you need two main components: the user interface (UI) and the server function. Here's a simple example:
library(shiny)
ui <- fluidPage(
titlePanel("Simple Shiny App"),
sidebarLayout(
sidebarPanel(
sliderInput("bins", "Number of bins:", min = 1, max = 50, value = 30)
),
mainPanel(
plotOutput("distPlot")
)
)
)
server <- function(input, output) {
output$distPlot <- renderPlot({
x <- faithful$eruptions
bins <- seq(min(x), max(x), length.out = input$bins + 1)
hist(x, breaks = bins, col = 'darkgray', border = 'white')
})
}
shinyApp(ui = ui, server = server)
This code creates a simple app that displays a histogram of the faithful dataset, with a slider to adjust the number of bins.
Deploying Your RShiny App
Once you've created your RShiny app, you can deploy it to share with others. RStudio offers a service called shinyapps.io where you can host your apps. To deploy your app, you need to install the rsconnect package and follow these steps:
- Install the rsconnect package:
install.packages("rsconnect")
- Load the package:
library(rsconnect)
- Deploy your app:
rsconnect::deployApp("path/to/your/app")
After deployment, your app will be accessible via a URL, allowing others to interact with your analysis and visualizations.
Conclusion
Mastering RStudio and RShiny opens up a world of possibilities in data analysis and visualization. By following these steps, you can go from writing simple R scripts to creating interactive web applications. Whether you're a beginner or looking to enhance your skills, RStudio provides the tools you need to succeed.
Start exploring and experimenting with RStudio today, and unlock new insights from your data.